Step by Step guide Seaborn for data analytics in Python
Seaborn is a powerful Python data visualization package based on Matplotlib. It is created specifically for data analytics and is especially beneficial for making attractive and instructive statistical visuals. Seaborn has a high-level interface for constructing several types of plots, making it simple to build visualizations for studying and analyzing your data.
Table of Contents
ToggleKey Features of Seaborn in python
High-level Interface:
Seaborn provides a concise and expressive API to create complex visualizations with just a few lines of code. It simplifies the process of creating common statistical plots, such as scatter plots, bar plots, histograms, box plots, violin plots, etc.
Statistical Plotting:
Seaborn comes with built-in functions for visualizing statistical relationships and distributions in your data. It allows you to create informative plots like regression plots, distribution plots, joint plots, pair plots, and more.
Attractive Improvements:
Seaborn enhances the visual appeal of plots by providing attractive default styles and color palettes. This saves you time and effort in fine-tuning the appearance of your visualizations.
Integration with Pandas:
Seaborn integrates seamlessly with Pandas DataFrames, allowing you to plot data directly from your datasets without extensive data manipulation.
Support for Categorical Data:
Seaborn handles categorical data well and can automatically adjust the plot aesthetics based on the data’s nature.
simple scatter plot using Seaborn
import seaborn as sns import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [5, 4, 3, 2, 1] # Create a scatter plot sns.scatterplot(x=x, y=y) # Show the plot plt.show()

sns.lineplot()
import seaborn as sns import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [5, 4, 3, 2, 1] # Create a line plot sns.lineplot(x=x, y=y) # Show the plot plt.show()
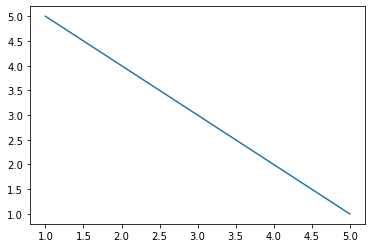
sns.relplot():
- A versatile function that can create various types of relational plots, including scatter plots, line plots, and more.
- It’s a higher-level function that allows you to easily switch between different plot types based on the data and context.
import seaborn as sns import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [5, 4, 3, 2, 1] # Create a scatter plot using relplot sns.relplot(x=x, y=y, kind='scatter') # Show the plot plt.show()

Categorical Plots:
Categorical plots in Seaborn are used to visualize the distribution and relationships between categorical variables. They are particularly useful for comparing data across different categories and identifying patterns or trends. Seaborn provides several functions to create categorical plots. Here are some of the key functions for categorical plots:
sns.barplot():
- Creates a bar plot to show the average value of a numerical variable for each category.
- The height of each bar represents the mean value, and error bars can be included to show confidence intervals or standard deviations.
- It’s useful for comparing the central tendency of a numerical variable across different categories
import seaborn as sns import matplotlib.pyplot as plt # Sample data categories = ['A', 'B', 'C', 'D'] values = [10, 15, 8, 12] # Create a bar plot sns.barplot(x=categories, y=values) # Show the plot plt.show()

sns.countplot()
import seaborn as sns import matplotlib.pyplot as plt # Sample data categories = ['A', 'B', 'A', 'C', 'A', 'B', 'D'] # Create a count plot sns.countplot(x=categories) # Show the plot plt.show()

sns.boxplot()
import seaborn as sns import matplotlib.pyplot as plt # Sample data categories = ['A', 'B', 'A', 'C', 'B', 'D'] values = [10, 15, 8, 12, 20, 5] # Create a box plot sns.boxplot(x=categories, y=values) # Show the plot plt.show()

sns.pointplot():
- Creates a point plot to show point estimates and confidence intervals for categorical data.
- It’s useful for visualizing the relationship between two categorical variables or a categorical variable and a numerical variable.
import seaborn as sns import matplotlib.pyplot as plt # Sample categories = ['A', 'B', 'A', 'C', 'B', 'D'] values = [10, 15, 8, 12, 20, 5] # Create a point plot sns.pointplot(x=categories, y=values) # Show the plot plt.show()
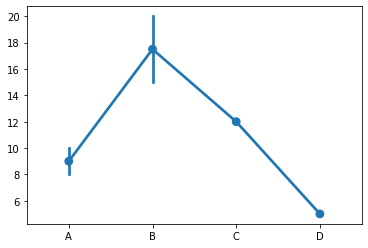
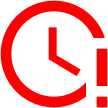
Time’s up